Jan 10, 2025
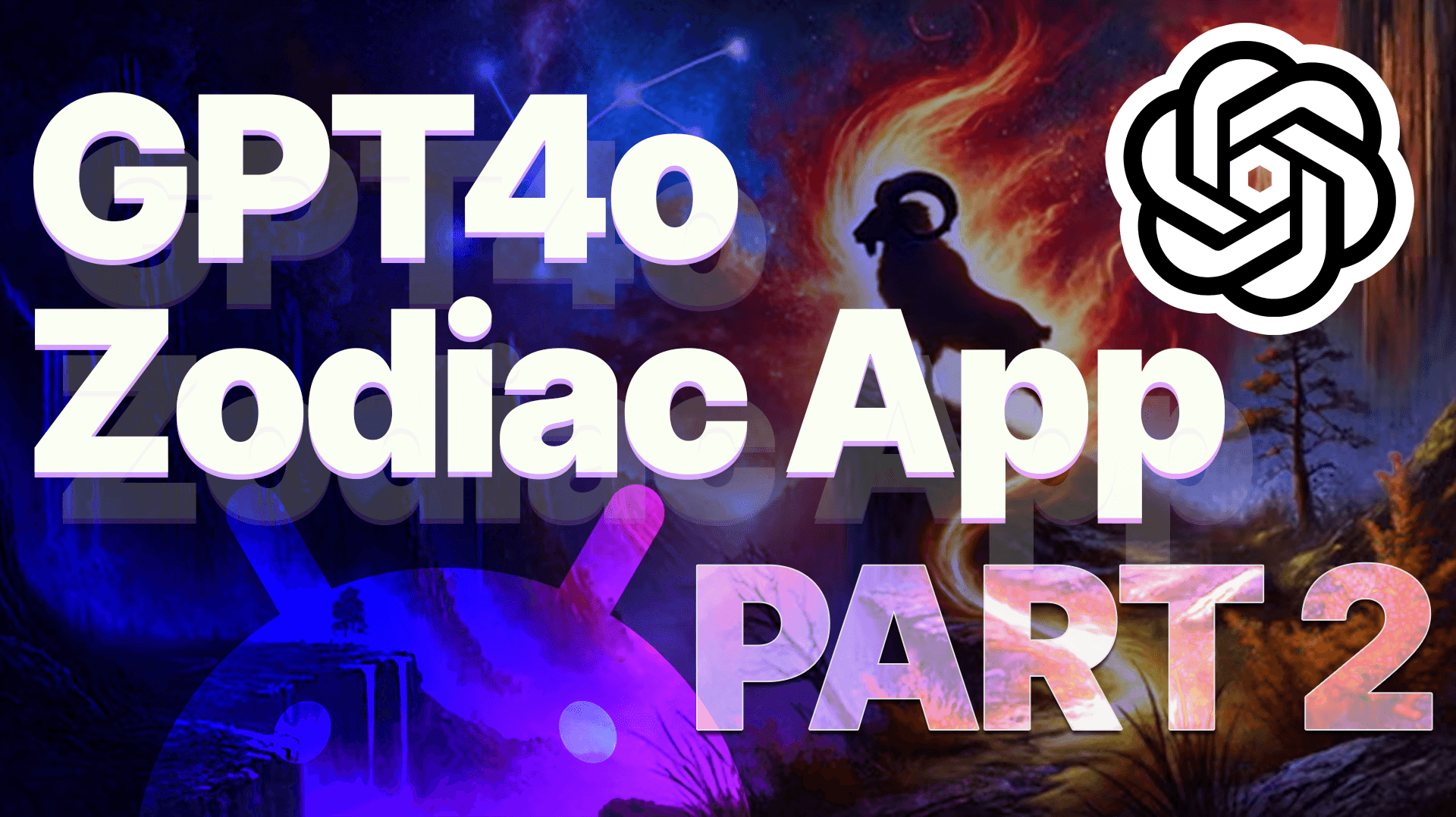
Welcome back to Part 2 of Generating a Zodiac Android App using GPT4o with Canvas. In the last tutorial, you learned how to utilize the new Canvas interface to create an Zodiac-themed Android app with a login screen, a list view, and a detail screen for each list item. You also engaged the use of DALL-E to generate images for our zodiac signs to bring some life to the app. Now, we are going to move on to building our backend by connecting to the OpenAI API to our daily horoscopes.
Creating the REST API Backend
One of the core components of our Zodiac app is the REST API backend that will generate and serve daily horoscopes. We’ll use Flask, a lightweight Python web framework, to create this API.
Let’s start by examining the basic structure of our Flask app:
This Flask app defines a single route /daily_horoscope
that accepts a zodiac_sign
parameter. It then uses the OpenAI API to generate a horoscope based on the provided zodiac sign. Create a directory named backend
and save this as an app.py
file there.
As is usually the case when dealing with API keys, you will need to make a config.py
file and save your OpenAI API key there. Feel free to check the OpenAI API Reference Docs for information if you are unfamiliar with the setup.
Run your app.py file from the console to give your code a test locally and see if it can produce a horoscope. If it worked, you should see a call response like this:
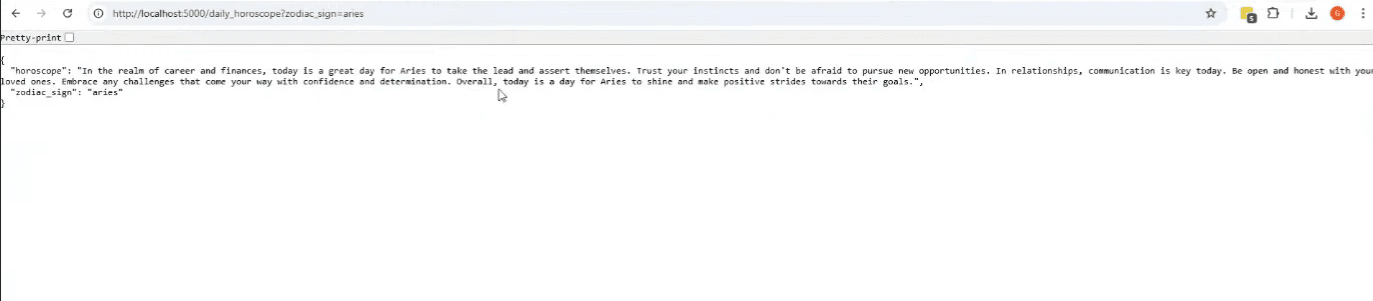
Hosting the API
To make our API accessible to our Android app, we need to host it somewhere. In this tutorial, we’ll use Glitch, a platform that allows for easy hosting of small web applications.
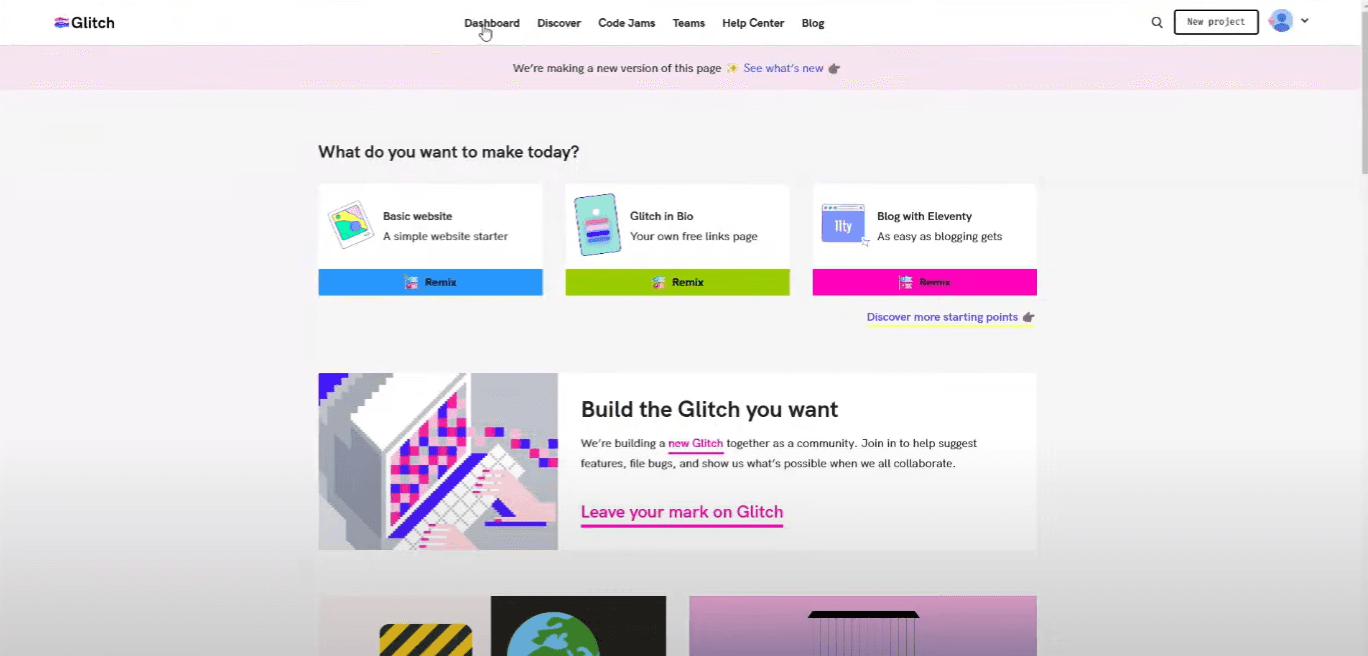
To deploy our Flask app on Glitch:
Create a new project on Glitch
Upload our Flask app files
Add a
requirements.txt
file with the necessary dependenciesopenai
config
flask
Add a
horoscope.yaml
(generated by ChatGPT) to give Glitch a blueprint of our appAdd a
start.sh
script to run the Flask app (copied from Glitch’s docs)
Once deployed, Glitch will provide us with a unique URL that we can use to access our API.
Integrating the API with Android
Now that our backend is set up, we need to integrate it into our Android app. We’ll use the OkHttp library to make HTTP requests to our API.
First, we need to add the OkHttp dependency to our build.gradle
file:
Make sure you add it to the app build.gradle
file and not the project one.
Back in OpenAI’s Canvas dashboard input the following prompt:
update ZodiacDetailActiity and activity_zodiac_detail so I can call an API, e.g. https://{{YOUR GLITCH APP URL}}?zodiac_sign=aries using OkHTTP to display a daily horoscope
Take note of the parameter after the ?
in url in the prompt above. We are essentially feeding the request the Aries zodiac sign.
Next, we’ll use that output to update our ZodiacDetailActivity
to make a request to our API and display the horoscope:
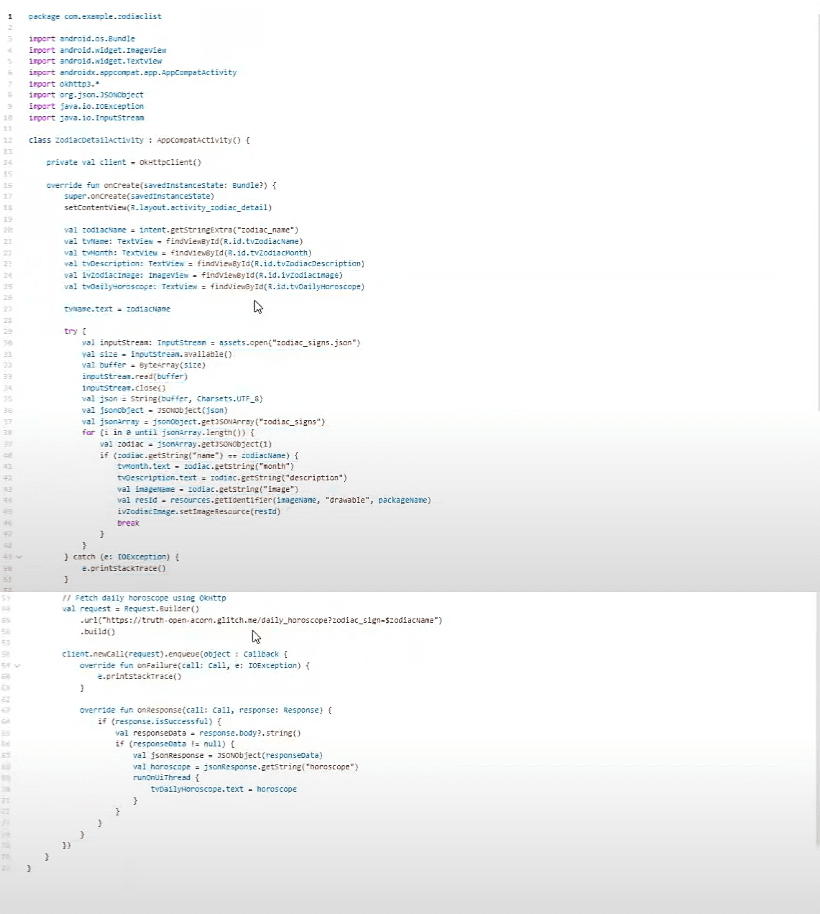
That’s making the request and updating the UI, but we also need to ensure our activity_zodiac_detail.xml has a textview
slot for the data to be placed. This should have also been generated with the last prompt, but if not, it never hurts to coax GPT again. The new section should look something like this:
Adding Internet Permission
To allow our app to make network requests, we need to add the internet permission to our AndroidManifest.xml
file:
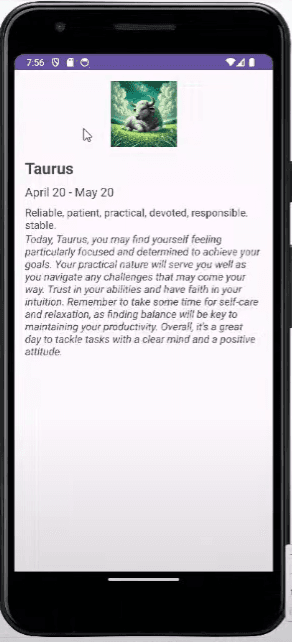
Congratulations!
Over the course of this small series, you've learned how to create a fully functional Zodiac app from scratch using GPT-4’s Canvas interface. You made a frontend using generated code and images and connected it to a dynamic backend using Flask and OkHttp. You’ve learned about the classic structure of an Android app, how to call the OpenAI API, and the simplicity of setting up a backend server on Glitch to manage the calls. You’ve done all of this without having to write tons of boilerplate code so you could focus on the plumbing and features of your app. It's time to start building that app you’ve been dreaming about with all that extra free time!
Additional Resources
For those interested in diving deeper into the topics covered in this tutorial, here are some helpful resources:
These resources provide additional context and code examples that can help you further enhance your Zodiac app or explore similar projects.